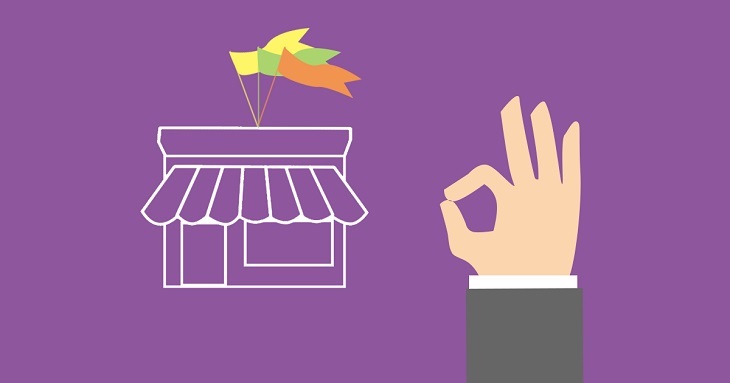
Understanding Remote Code Execution in Web Security: A Deep Dive with PHP Examples
In the world of web security, one of the most critical and dangerous vulnerabilities is remote code execution (RCE). It allows attackers to execute arbitrary code on a targeted server or application, enabling them to control the system and potentially gain unauthorized access. In this blog post, we will explore remote code execution in web security, with a specific focus on PHP, one of the most widely used programming languages for web development.
What is Remote Code Execution (RCE)?
Remote Code Execution refers to the ability of an attacker to execute arbitrary code on a target system or application remotely, typically over the internet. It occurs when an application fails to properly validate or sanitize user input and allows the execution of unintended code. The consequences of RCE can be severe, ranging from data breaches and unauthorized access to complete compromise of the target system.
Understanding RCE in PHP
PHP is a popular server-side scripting language used for web development. It offers various features and functions that, if not carefully used, can introduce RCE vulnerabilities. Let’s understand how an RCE vulnerability can be exploited in a PHP application using an example.
Consider a PHP application that allows users to upload profile pictures. The application might have a code snippet like this:
<?php
$targetDir = "uploads/";
$targetFile = $targetDir . basename($_FILES["profilePicture"]["name"]);
$uploadOk = 1;
$imageFileType = strtolower(pathinfo($targetFile, PATHINFO_EXTENSION));
// Check if image file is a actual image or fake image
if (isset($_POST["submit"])) {
$check = getimagesize($_FILES["profilePicture"]["tmp_name"]);
if ($check !== false) {
$uploadOk = 1;
} else {
$uploadOk = 0;
}
}
// Check file size
if ($_FILES["profilePicture"]["size"] > 500000) {
$uploadOk = 0;
}
// Allow certain file formats
if ($imageFileType != "jpg" && $imageFileType != "png" && $imageFileType != "jpeg" && $imageFileType != "gif") {
$uploadOk = 0;
}
// Check if $uploadOk is set to 0 by an error
if ($uploadOk == 0) {
echo "Sorry, your file was not uploaded.";
} else {
if (move_uploaded_file($_FILES["profilePicture"]["tmp_name"], $targetFile)) {
echo "The file " . basename($_FILES["profilePicture"]["name"]) . " has been uploaded.";
// Display the uploaded profile picture
echo "<img src='" . $targetFile . "'>";
} else {
echo "Sorry, there was an error uploading your file.";
}
}
?>
While this code snippet ensures some basic validation on the uploaded file, it is still vulnerable to RCE. An attacker could exploit this vulnerability by uploading a malicious PHP file instead of an image file. The uploaded file can then be executed by constructing a specific request to the PHP server.
For example, if the attacker uploads a file named “payload.php” containing malicious code, they can execute it by accessing the following URL: http://example.com/uploads/payload.php
. This allows the attacker to run arbitrary code on the server, potentially leading to a complete compromise of the system.
Mitigating Remote Code Execution Vulnerabilities
To protect your applications from remote code execution vulnerabilities, it’s crucial to follow secure coding practices and implement proper security measures. Here are some mitigation techniques that can help prevent RCE attacks:
1. Input Validation and Sanitization
Always validate and sanitize user input to ensure it conforms to expected formats and patterns. Use appropriate validation and sanitization methods based on the data type to prevent harmful code from being executed. For example, when accepting file uploads, validate the file type, size, and perform server-side file type checking.
In our PHP example, you could use a library like exif_imagetype()
to check if an uploaded file is a legitimate image, rather than just relying on the file extension. Additionally, you should consider storing uploaded files outside the web root directory to prevent direct access.
2. Principle of Least Privilege
Follow the principle of least privilege when granting permissions and privileges to your web application and server infrastructure. Restrict the execution capabilities and reduce the attack surface by using limited user accounts with minimal permissions. Regularly review and remove unnecessary privileges.
3. Regular Security Updates
Keep your server software, frameworks, and libraries up to date with the latest security patches. Regularly check for updates and apply them promptly to address any known vulnerabilities. Stay informed about any security advisories related to the components used in your application.
4. Use Web Application Firewalls (WAF)
Implement a Web Application Firewall as an additional layer of defense. WAFs can help detect and block common attacks, including RCE attempts, by inspecting the traffic and blocking any requests that match known attack patterns. Choose a WAF solution that is well-maintained, regularly updated, and configured to meet your application’s specific needs.
5. Secure Code Review and Penetration Testing
Perform regular code reviews by experienced developers to identify potential security vulnerabilities. Integrate secure coding practices into your development process and use static code analysis tools to catch common coding errors and detect potential issues. Additionally, conduct penetration testing to identify any vulnerabilities that might have been missed during the development process.
Conclusion
Remote Code Execution (RCE) vulnerabilities pose a significant threat to the security of web applications. By understanding how RCE can occur in PHP applications and implementing the recommended mitigation techniques, you can significantly reduce the risk of exploitation. Remember to always prioritize the security of your applications, stay updated with the latest security practices, and follow a defense-in-depth approach to protect against remote code execution attacks.
Borealis Bytes offers top-notch auditing and consulting services to help secure your application landscape. Contact us today for a free consultation and let’s discuss how we can help strengthen your application security together.