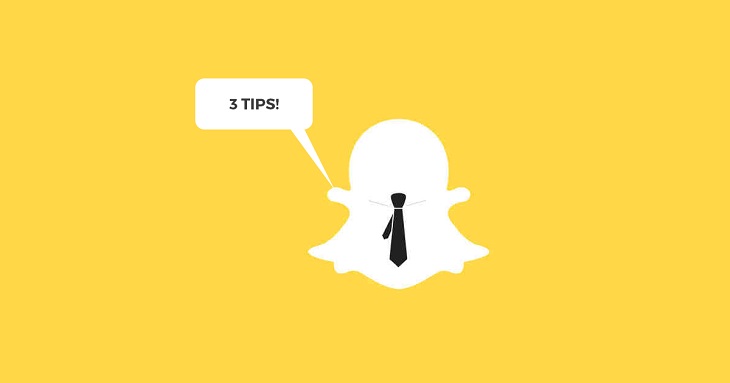
Introduction to Dompurify: Securely Sanitize User Input in JavaScript
Introduction to Dompurify: Securely Sanitize User Input in JavaScript
As web developers, one of our top priorities is to ensure the security of the applications we build. It is essential to protect user data and prevent malicious attacks such as Cross-Site Scripting (XSS) vulnerabilities. One way to achieve this is by properly sanitizing user input to remove any potentially harmful content. In this blog post, we will explore the concept of sanitization and discuss an excellent tool called Dompurify that enables us to securely sanitize user input in JavaScript.
What is Sanitization?
Sanitization is the process of cleaning and validating user input to ensure it adheres to the expected format and does not contain any potentially harmful code. By properly sanitizing user input, we can reduce the risk of XSS attacks, where an attacker injects malicious code into our application through user-provided content.
When sanitizing user input, we need to carefully evaluate and remove any HTML tags, attributes, or JavaScript code that could pose a security threat. It is crucial not to trust user input blindly and to be aware of the potential risks.
Introducing Dompurify
Dompurify is a JavaScript library that provides a simple and effective method for sanitizing user input. It uses a combination of safe DOM methods and regular expressions to remove any potentially dangerous code from the input while preserving its intended functionality.
Dompurify offers a robust and reliable solution for sanitizing user input, making it an essential tool for web developers concerned about application security. Now, let’s explore how Dompurify works and how we can use it in our JavaScript code.
How Does Dompurify Work?
Dompurify works by parsing the provided HTML string, identifying any potentially dangerous elements or attributes, and removing them from the output. It uses a whitelist approach, meaning that it only allows specific elements and attributes that are considered safe.
Here’s an example code snippet that demonstrates how to use Dompurify:
import DOMPurify from 'dompurify';
const userInput = '<script>alert("XSS attack!");</script><p>Some safe content</p>';
const sanitizedInput = DOMPurify.sanitize(userInput);
console.log(sanitizedInput);
In the example above, we import the Dompurify library using an ES6 module import statement. We then define a variable userInput
containing a string that represents user input, which includes an embedded JavaScript code for an XSS attack and some safe content.
Next, we use the DOMPurify.sanitize()
method provided by Dompurify to sanitize the user input. The method returns a sanitized version of the input string, removing the malicious JavaScript code while preserving the safe content.
Finally, we log the sanitized input to the console, which will output:
<p>Some safe content</p>
As you can see, the unsafe script tag has been removed, and only the safe content remains.
Advanced Usage of Dompurify
Dompurify provides additional options and methods for more advanced use cases. Let’s explore some of these features below:
Configuring a Custom Sanitizer Function
Dompurify allows you to define a custom sanitizer function to further customize the sanitization process. This function is called for each HTML element to determine whether it should be allowed or not.
Here’s an example of how to configure a custom sanitizer function:
import DOMPurify from 'dompurify';
const userInput = '<img src="x" onerror="alert(\'XSS attack!\');" />';
const sanitizedInput = DOMPurify.sanitize(userInput, {
USE_CUSTOM_SPECS: true,
ADD_TAGS: ['img'],
ALLOWED_ATTR: ['src'],
FORBID_ATTR: ['onerror'],
FORBID_TAGS: [],
});
console.log(sanitizedInput);
In this example, we define a userInput
string that includes an image tag with an invalid src
attribute and an onerror
attribute with an XSS attack. We configure Dompurify to use a custom sanitizer function by setting the USE_CUSTOM_SPECS
option to true
. We then specify the allowed and forbidden tags and attributes using the corresponding options.
The output of this code snippet will be:
<img src="x">
As you can see, only the src
attribute is preserved, while the onerror
attribute and the XSS attack are removed.
Sanitizing HTML Fragments
Dompurify also provides a method called sanitizeDOMFragment()
that allows you to sanitize a fragment of HTML. This can be useful when working with dynamic content, such as injecting HTML code into the DOM.
Here’s an example demonstrating the usage of sanitizeDOMFragment()
:
import DOMPurify from 'dompurify';
const htmlFragment = '<div><p>Some safe content</p></div>';
const sanitizedFragment = DOMPurify.sanitizeDOMFragment(htmlFragment);
console.log(sanitizedFragment);
In this example, we define an HTML fragment using a <div>
element containing some safe content. We then use the sanitizeDOMFragment()
method to sanitize the HTML fragment. The method returns a sanitized version of the HTML fragment, removing any potentially dangerous code.
The output of this code snippet will be:
<div><p>Some safe content</p></div>
As you can see, the HTML fragment remains unchanged since it only contains safe content.
Conclusion
In this blog post, we explored the concept of sanitization and discussed the importance of properly sanitizing user input to ensure application security. We introduced Dompurify, a powerful JavaScript library that enables us to securely sanitize user input.
We learned how Dompurify works by parsing HTML strings, identifying potentially dangerous elements and attributes, and removing them from the output. We also explored advanced features, such as configuring a custom sanitizer function and sanitizing HTML fragments.
By incorporating Dompurify into our JavaScript code, we can significantly reduce the risk of XSS attacks and enhance the security of our applications.
Borealis Bytes offers top-notch auditing and consulting services to help secure your application landscape. Contact us today for a free consultation and let’s discuss how we can help strengthen your application security together.